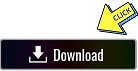
Lock readLock()returns the lock that's used for reading.ReadWriteLock declares methods to acquire read or write locks: The read lock may be simultaneously held by multiple threads as long as there is no write. In addition to the Lock interface, we have a ReadWriteLock interface that maintains a pair of locks, one for read-only operations and one for the write operation.
#LOCK QUEUE CODE#
void unlock() unlocks the Lock instance.Ī locked instance should always be unlocked to avoid deadlock condition.Ī recommended code block to use the lock should contain a try/catch and finally block: Lock lock =. boolean tryLock(long timeout, TimeUnit timeUnit) – This is similar to tryLock(), except it waits up the given timeout before giving up trying to acquire the Lock. It attempts to acquire the lock immediately, return true if locking succeeds. boolean tryLock()– This is a nonblocking version of lock() method. void lockInterruptibly() – This is similar to the lock(), but it allows the blocked thread to be interrupted and resume the execution through a thrown. If the lock isn't available, a thread gets blocked until the lock is released.
void lock() – Acquire the lock if it's available. The Lock API provides a method lockInterruptibly() that can be used to interrupt the thread when it's waiting for the lock. A thread that is in “waiting” state to acquire the access to synchronized block can't be interrupted. This reduces blocking time of thread waiting for the lock. The thread acquires lock only if it's available and not held by any other thread. A thread gets blocked if it can't get an access to the synchronized block.
It makes sure that the longest waiting thread is given access to the lock. We can achieve fairness within the Lock APIs by specifying the fairness property. Any thread can acquire the lock once released, and no preference can be specified. A s ynchronized block doesn't support the fairness.We can have Lock APIs lock() and unlock() operation in separate methods. A synchronized block is fully contained within a method.
There are a few differences between the use of synchronized block and using Lock APIs: